Why Your Code Doesn’t Work on Fridays: Debugging with a Cup of Coffee
Friday is here. The code that worked yesterday is spewing errors more quickly than you can Google them, you're exhausted, and the team is eager for the weekend. On a Friday, debugging is like attempting to solve a Rubik's Cube while wearing a blindfold; everything is disjointed and illogical. What makes debugging more difficult toward the end of the week, then? And how can you make it better, or at least make it work?
Let's examine some typical mistakes, psychological traps, and environmental elements that can undermine your debugging efforts, as well as how a cup of tea or coffee can occasionally help.
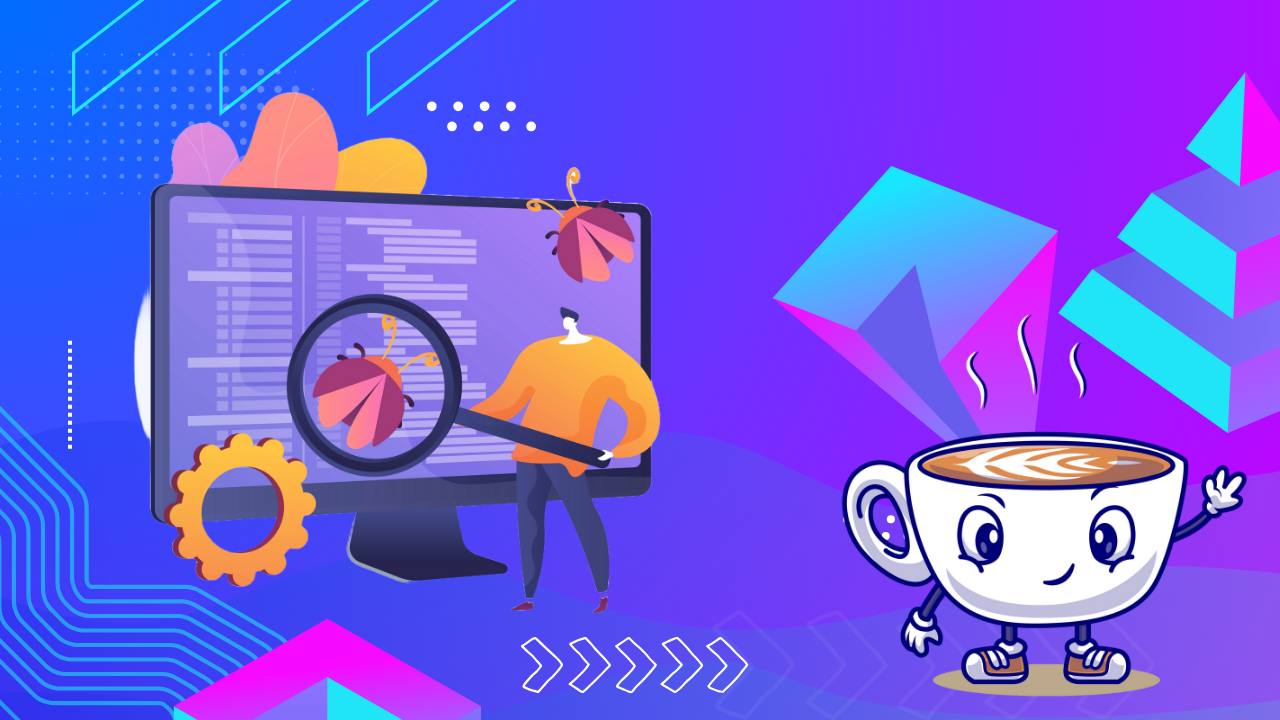
#
The Usual Suspects: Common Friday Code Failures#
1. The "Last-Minute Change" SyndromeJust one quick tweak before the weekend" is always the first line. Even minor codebase modifications, like as changing a variable name or modifying a query, can have unanticipated consequences. Unrelated system components can be broken by seemingly innocuous things.
Tip: Make sure you adhere to version control. Make frequent modifications and reserve Fridays for documentation or low-risk, minor activities.
#
2. Stale Development EnvironmentThe staging or production servers might not be in sync with your local environment. Head-scratching problems might result from obsolete configurations, missing dependencies, or even forgotten npm install
scripts.
Tip: Run a clean environment setup (Docker Compose documentation) to ensure you're debugging in a reliable sandbox.
#
3. Over-Optimizing Without ContextFriday is infamous for its hasty optimization efforts. You rewrite, modify performance settings, or alter an algorithm without conducting adequate testing. Your flawlessly functioning code suddenly becomes slower or, worse, stops working altogether.
Tip: Save optimizations for midweek when you have time to test thoroughly. Friday is for maintenance, not reinvention.
#
4. Ignoring Logs and Error MessagesIt's easy to glance past confusing stack traces or error logs in your haste to complete chores. Friday debugging necessitates a laser-like attention on logs, yet "I'll figure it out later" becomes a motto.
Tip: Set up structured logging and use tools like grep, jq, or your IDE's log viewer to quickly narrow down the issue.
#
Debugging: It’s Not Just About CodeThe quality of your environment and mindset is just as important to debugging success as the quality of your code. Here are some ways that outside influences contribute to Friday's difficulties:
#
1. Mental FatigueYour mind had been working nonstop for days by Friday. Deep concentration, pattern identification, and logical reasoning are all necessary for debugging, and these skills deteriorate with mental fatigue. Solution: Move away from the screen. Stretch, go on a walk, or get that coffee that could save your life. You can view the issue more clearly after a quick reset..
#
2. Poor Workspace SetupA messy workstation or a disorganized IDE might quietly exacerbate mental overload. Your mind frequently mimics an unorganized environment. Solution: Spend 10 minutes tidying your workspace. Close irrelevant browser tabs, clean up open files in your editor, and ensure you’re focusing on one problem at a time.
#
3. Overloaded ToolsSometimes your tools, not you, are the problem. Friction might be introduced by outdated plugins, improperly configured linters, or bloated environments. Solution: Review your development environment. Keep your tools updated and lightweight, and invest time in learning productivity-boosting shortcuts or features.
#
4. The "Weekend Is Calling" EffectIt's difficult to avoid taking shortcuts when the finish line is in view. Missed defects, untested test cases, and unfinished solutions are frequently the results of the "just ship it" mentality. Solution: Write everything down. Document the problem, the potential fixes you tried, and any outstanding questions. Future you (on Monday) will thank you.
#
The Coffee Debugging RitualDebugging is a ritual rather than merely a talent. It might be really beneficial to give your problem-solving process structure, particularly on Fridays. Here is a basic debugging procedure fueled by coffee:
#
1. Brew Your Coffee (or Tea)Take advantage of the brief brewing time to clear your head. Take a deep breath, clear your head, and consider the issue from all angles.
#
2. Define the ProblemBefore touching the keyboard, ask yourself:
- What exactly is broken?
- What changed recently?
- Can I reproduce this consistently?
#
3. Divide and ConquerDivide the issue into manageable chunks. Concentrate on a single API call, function, or module at a time.
#
4. Read the LogsBring coffee so you may properly examine the logs. Pay attention to unexpected inputs or outputs, stack traces, and timestamps.
#
5. Rubber Duck ItTell a rubber duck (what is rubber duck debugging?) or a coworker about the issue. Putting the problem into words frequently results in breakthroughs.
#
6. Know When to StopIf the issue seems unsolvable, put your newfound knowledge in writing and come back to it on Monday. What Friday couldn't solve is frequently resolved by new eyes and a renewed thinking.
#
Final ThoughtsFriday debugging doesn't have to be a punishment. You can overcome even the most difficult obstacles without losing your sanity if you have the correct attitude, the appropriate equipment, and a consistent coffee routine. Keep in mind that all programmers get days off. Treat yourself with kindness, take breaks, and remember that Monday offers another opportunity to make amends for Friday's failure. Cheers to stronger coffee, better Fridays, and fewer bugs! ☕