Handling Errors in C# the Easy Way
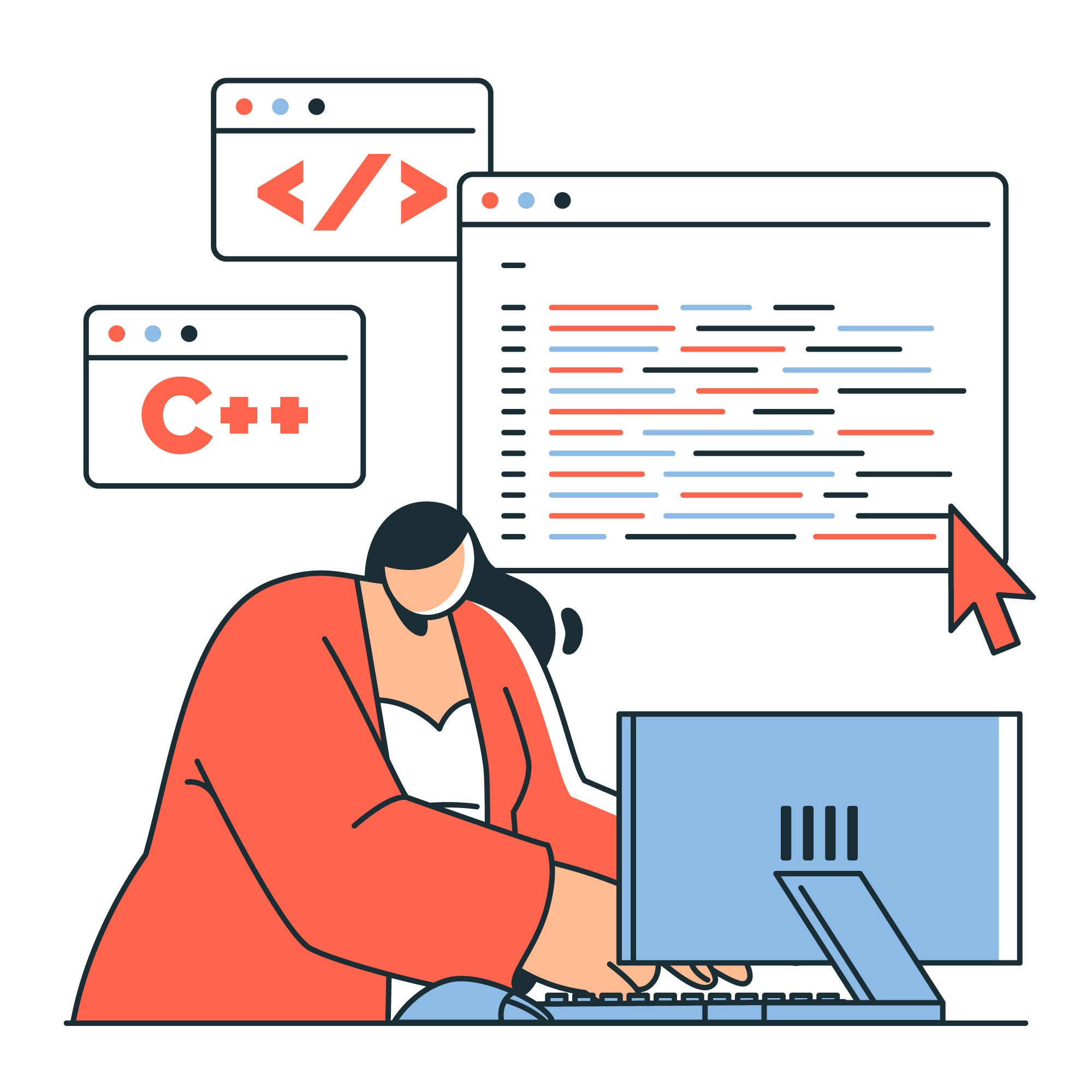
You are aware that things don't always go as planned if you have ever dealt with C# or any type of online API. There are instances when you get strange JSON, when a field is missing, and when—well—things just break. The good news is that you don't have to let your app crash and burn because of such problems. We can apprehend them, record them, and continue on.
I'll demonstrate how to use a custom error response object to handle errors in C# in this post. It's similar to building a safety net for your software so that it doesn't go into full panic mode when something goes wrong.
#
Why Do We Care About Custom Error Responses?It's not always sufficient to simply log or print an error to the console when it occurs in your application. You may want to provide more information about the issue, track several faults that occur simultaneously, or simply deliver a kind, easy-to-read message to the user. A custom error answer can help with that.
With a custom error response object, you can:
- Track different types of errors.
- Organize your errors into categories (so you know if it's a JSON issue, a database issue, etc.).
- Handle the error, log it, and then move on without crashing the app.
#
Setting Up Our Custom Error Object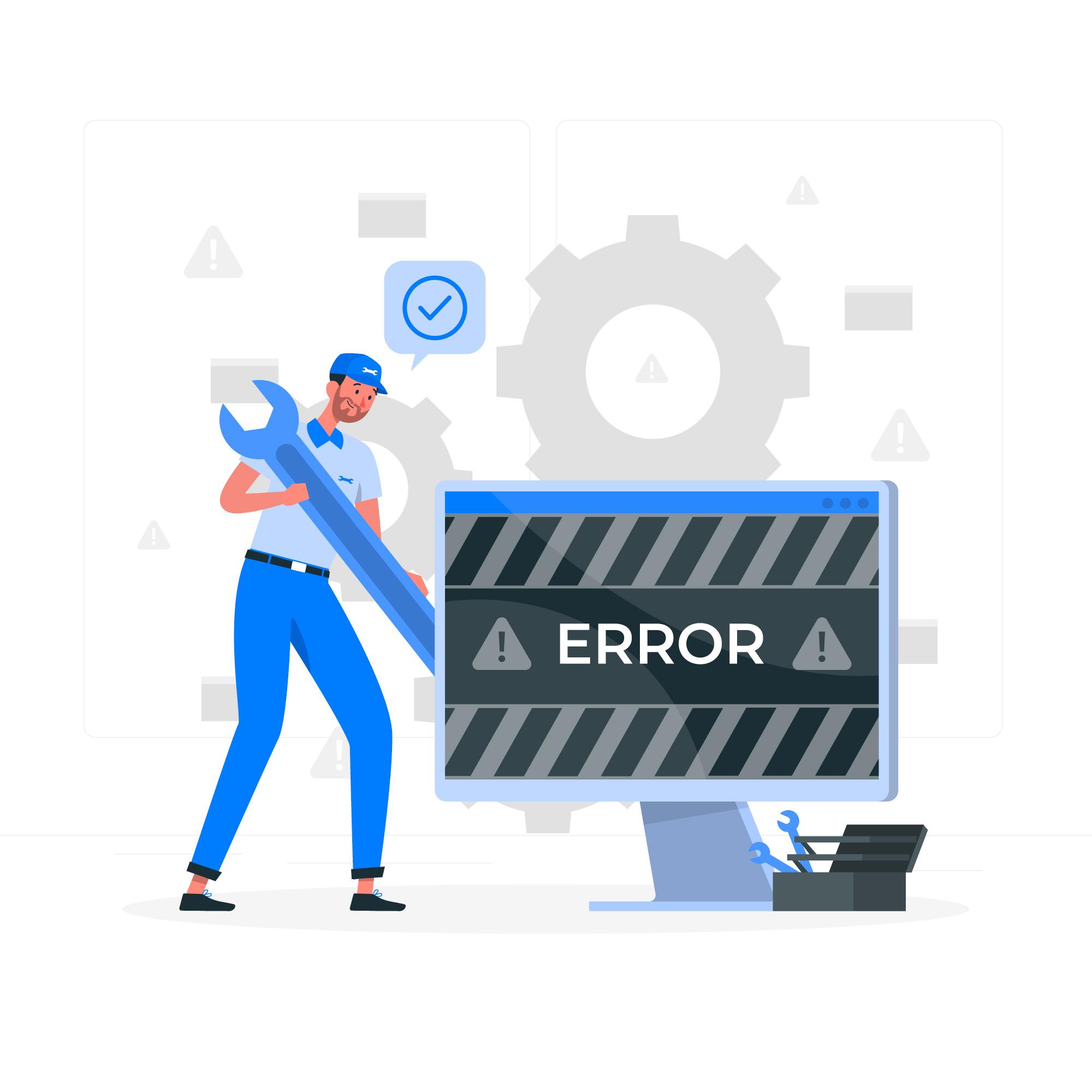
Let's start by setting up a basic error response object. This will hold our error messages in a dictionary, so we can track multiple types of errors.
Here's how you can do it:
- Message: This is just a generic message about what went wrong.
- Errors: This is a dictionary that'll hold all the different errors. Each key will represent an error type (like "JsonError" or "GeneralError"), and the value will be a list of error messages. This way, we can keep things organized.
#
Deserializing JSON and Handling ErrorsLet's say you're deserializing some JSON data, but there's a chance it could fail. Instead of letting the program crash, we can catch that error, store the details in our custom error response, and continue running. Here's how to do it:
#
What's Going On Here?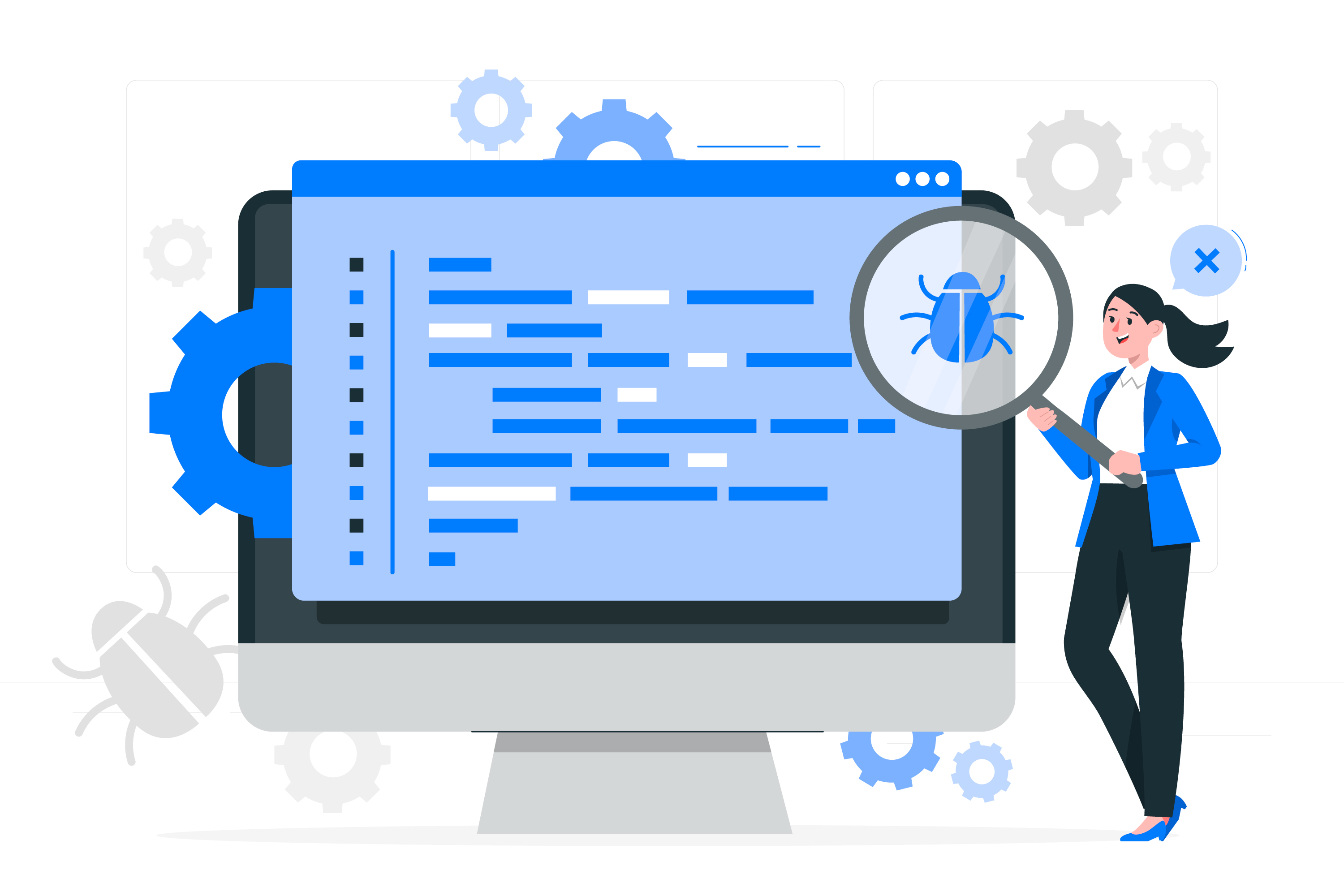
- Deserialization: We attempt to create our
ErrResponse
object from the JSON. Fantastic if it does. If not, the error is detected. - Catching JSON Errors: If the JSON is incorrect, we detect it and use a
JsonError
value to add it to ourErrors
dictionary. The error notice and stack trace are then displayed for simpler debugging. - General Error Handling: We detect and record any unexpected events (such as database problems or network failures) under the
GeneralError
key. - Program Doesn't Crash: The software continues to operate after the problem has been handled. Thus, without ruining anything, you can log issues, alert someone, or simply go on.
#
Why This Is Useful- It Keeps Things Neat: We store errors in an organised manner that makes it simple to see what's wrong, as opposed to simply throwing them around.
- Multiple Errors? No Problem: We don't have to overwrite or overlook anything when we use a dictionary to track numerous faults at once.
- No App Crashes: In the event that something breaks, the program continues to operate. You recognise the mistake, correct it, and move on with your life.
#
ConclusionError management doesn't have to be difficult. You may effortlessly handle failures, record crucial information, and maintain the functionality of your program by utilising a custom error response object in C#. There are ways to deal with issues like a broken JSON string or an unplanned crash without everything exploding.
Therefore, bear in mind to identify the mistake, manage it politely, and continue working on your program the next time something goes wrong.
If you're looking for cutting-edge features for cloud deployment, check out what Oikos by Nife has to offer.