How to Convert PDF.js to UMD Format Automatically Using Babel
If you've used PDF.js in JavaScript projects, you might have noticed the pdfjs-dist
package provides files in ES6 module format (.mjs
). But what if your project needs UMD-compatible .js
files instead?
In this guide, I'll show you how to automatically transpile PDF.js from .mjs
to UMD using Babel—no manual conversion required.
#
Why UMD Instead of ES6 Modules?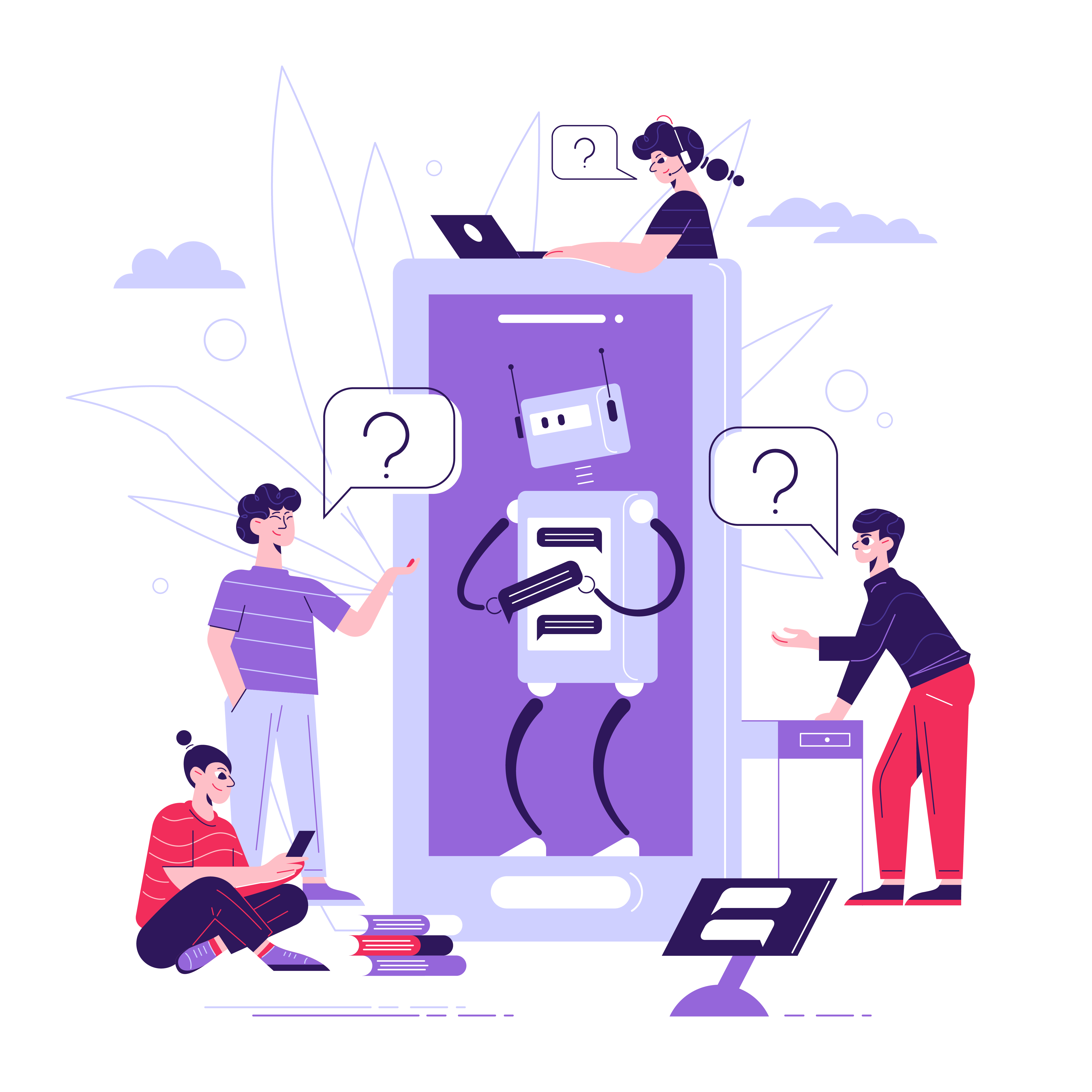
Before we dive in, let’s clarify the difference:
ES6 Modules (.mjs
)
- Modern JavaScript standard
- Works natively in newer browsers and Node.js
- Uses
import/export
syntax
UMD (.js
)
- Works in older browsers, Node.js, and AMD loaders
- Better for legacy projects or bundlers that don’t support ES6
If your environment doesn’t support ES6 modules, UMD is the way to go.
See how module formats differ →
#
The Solution: Automate Transpilation with Babel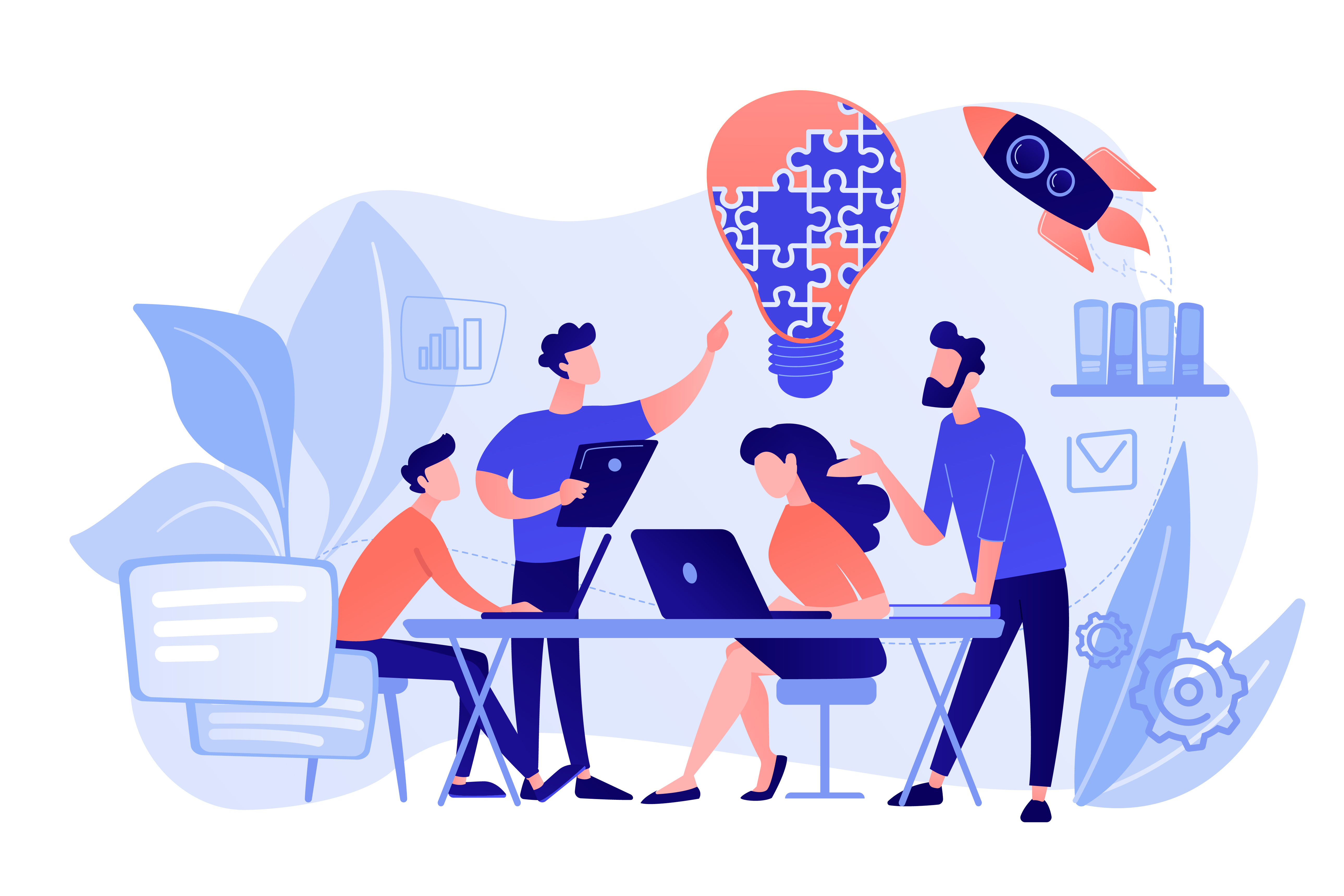
Instead of searching for pre-built UMD files (which may not exist), we’ll use Babel to convert them automatically.
#
Step 1: Install Babel & Required PluginsFirst, install these globally (or locally in your project):
@babel/cli
→ Runs Babel from the command line@babel/preset-env
→ Converts modern JS to compatible code@babel/plugin-transform-modules-umd
→ Converts modules to UMD format
For more on Babel configurations, check out the official Babel docs.
#
Step 2: Create the Transpilation ScriptSave this as transpile_pdfjs.sh
:
Merge multiple PDFs into a single file effortlessly with our Free PDF Merger and Split large PDFs into smaller, manageable documents using our Free PDF Splitter.
#
Step 3: Run the ScriptMake it executable:
Execute it:
#
What’s Happening?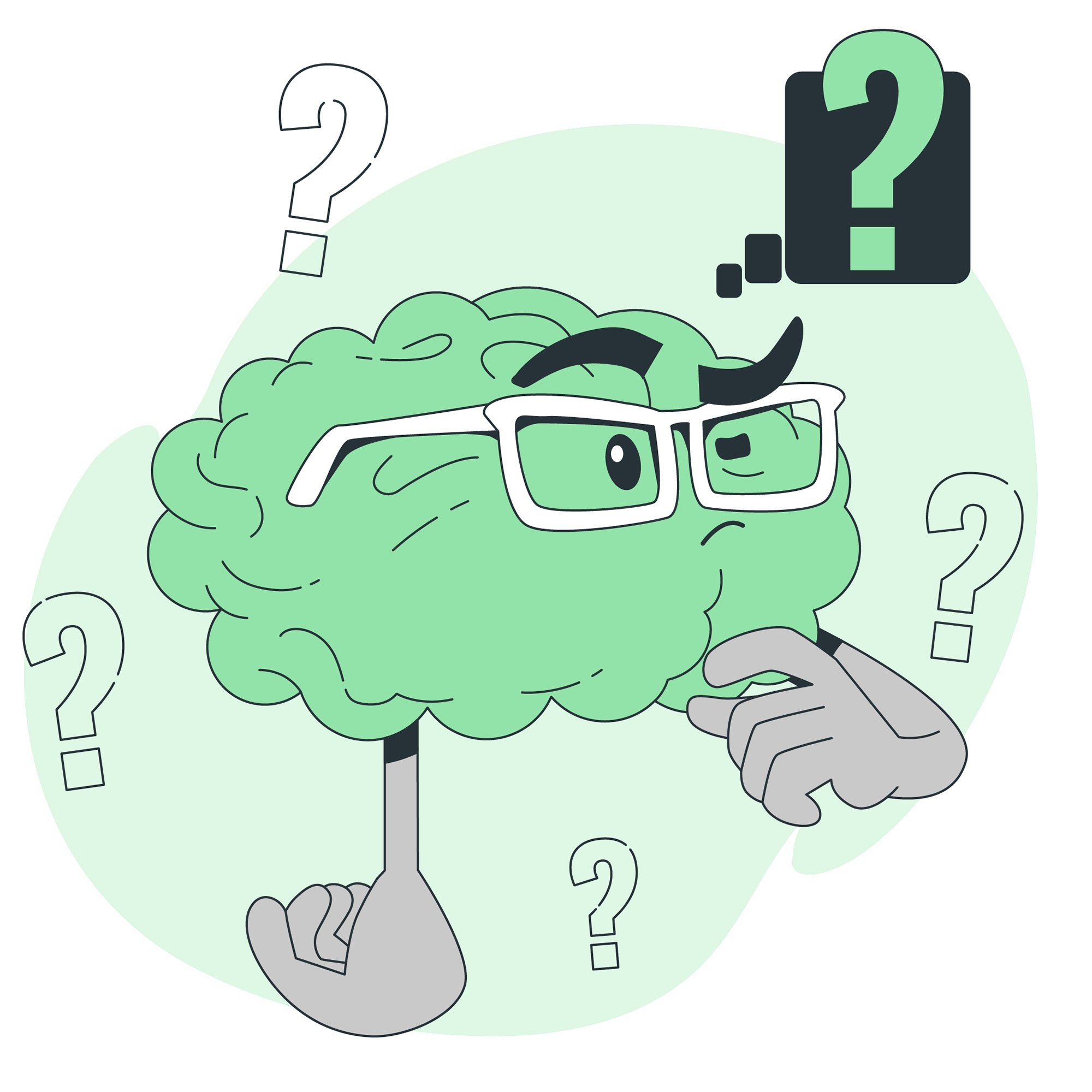
✔ Checks for Babel → Ensures the tool is installed.
✔ Creates a umd
folder → Stores the converted .js
files.
✔ Transpiles .mjs
→ UMD → Uses Babel to convert module formats.
✔ Skips minified files → Avoids re-processing .min.mjs
.
Want to automate your JS build process further? Check out this BrowserStack.
#
Final ThoughtsNow you can use PDF.js in any environment, even if it doesn’t support ES6 modules!
🔹 No manual conversion → Fully automated.
🔹 Works with the latest pdfjs-dist
→ Always up-to-date.
🔹 Reusable script → Run it anytime you update PDF.js.
And if you want to bundle your PDF.js output, Rollup’s guide to output formats is a great next read.
Next time you need UMD-compatible PDF.js, just run this script and you’re done!
Simplify the deployment of your Node.js applications Check out this nife.io.