Comparing and Debugging ORA vs. PG Stored Procedures: A Generic Example
Stored procedures are an essential component of relational databases that help with logic encapsulation, performance improvement, and process automation. Both Oracle (ORA) and PostgreSQL (PG) include stored procedure functionality, however they are very different from each other. The distinctions between PG and ORA stored procedures as well as debugging techniques will be covered in this blog post. A simple general example will be provided to demonstrate these distinctions.
#
1. Stored Procedures in Oracle vs. PostgreSQL: Key Differences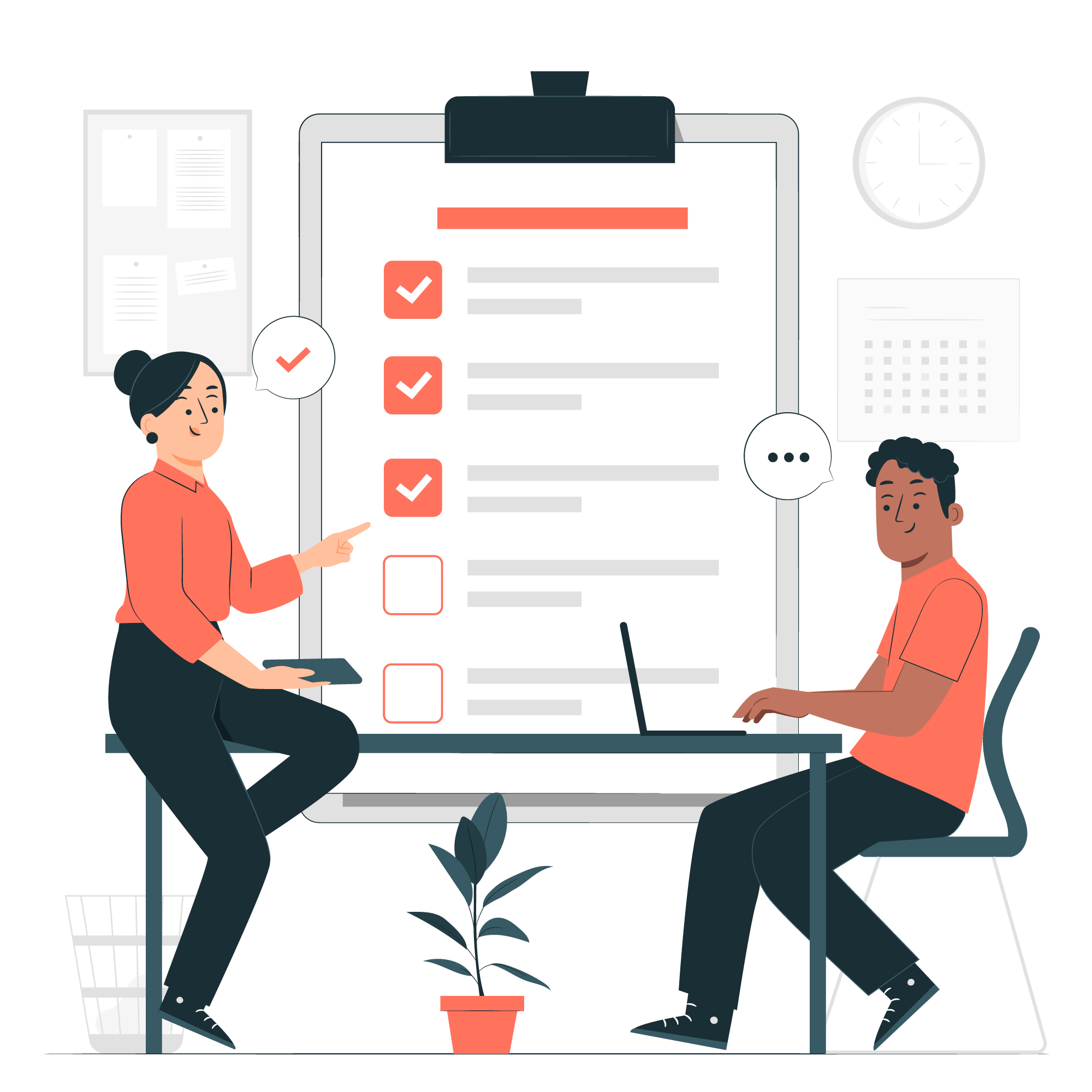
Despite being compatible with both ORA and PG, stored procedures differ significantly in terms of syntax, functionality, and debugging techniques. Let's look at the primary differences:
#
Syntax Differences#
Oracle (ORA):Oracle stored procedures are typically created using the CREATE PROCEDURE
command and utilize PL/SQL, a procedural extension of SQL. They explicitly use IN
, OUT
, and IN OUT
parameters and are wrapped in a BEGIN...END
block.
#
PostgreSQL (PG):PostgreSQL uses PL/pgSQL for stored procedures and functions, which is similar to Oracles PL/SQL but differs in syntax and capabilities. In PG:
- Stored procedures are created using
CREATE PROCEDURE
(introduced in version 11). - Functions are created using
CREATE FUNCTION
. - Unlike Oracle, PG does not support
IN OUT
parameters.
#
Example: A Generic Stored ProcedureThe following example determines whether a case belongs to a particular receiver type and sets an output flag appropriately.
#
Oracle (ORA) Example#
PostgreSQL (PG) Example#
Key Differences in Syntax- Procedure Declaration: Oracle explicitly defines
IN, OUT, IN OUT
parameter modes, whereas PostgreSQL only usesIN
orOUT
. - Exception Handling: Oracle uses
EXCEPTION
blocks withWHEN OTHERS THEN SQLERRM
to capture errors, while PostgreSQL mainly relies onRAISE EXCEPTION
. - Logic for No Data: Oracle explicitly checks for
NULL
, while PostgreSQL uses theFOUND
condition.
#
2. Debugging Stored Procedures in ORA vs. PG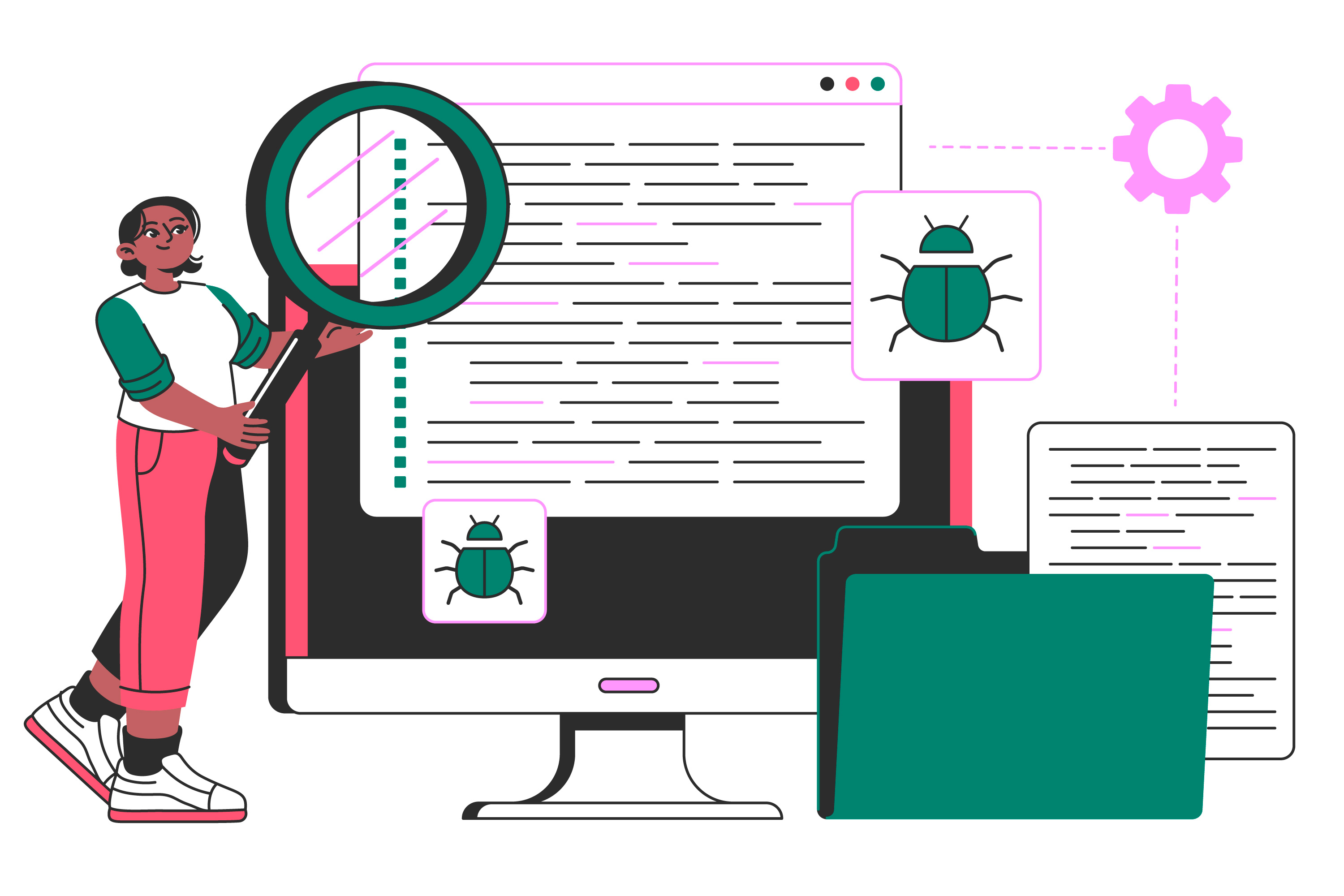
#
Oracle (ORA) Debugging- Use
DBMS_OUTPUT.PUT_LINE
to output debugging messages. - Handle exceptions using
WHEN OTHERS THEN
and log errors to custom tables. - Use Oracle SQL Developer for interactive debugging.
DBMS_OUTPUT
#
Example: Debugging with #
PostgreSQL (PG) Debugging- Use
RAISE NOTICE
for debugging output. - Handle exceptions using
RAISE EXCEPTION
and log errors to a dedicated table. - PostgreSQL lacks an integrated debugger like Oracle SQL Developer, so debugging relies on logging and manual testing.
RAISE NOTICE
#
Example: Debugging with #
3. Conclusion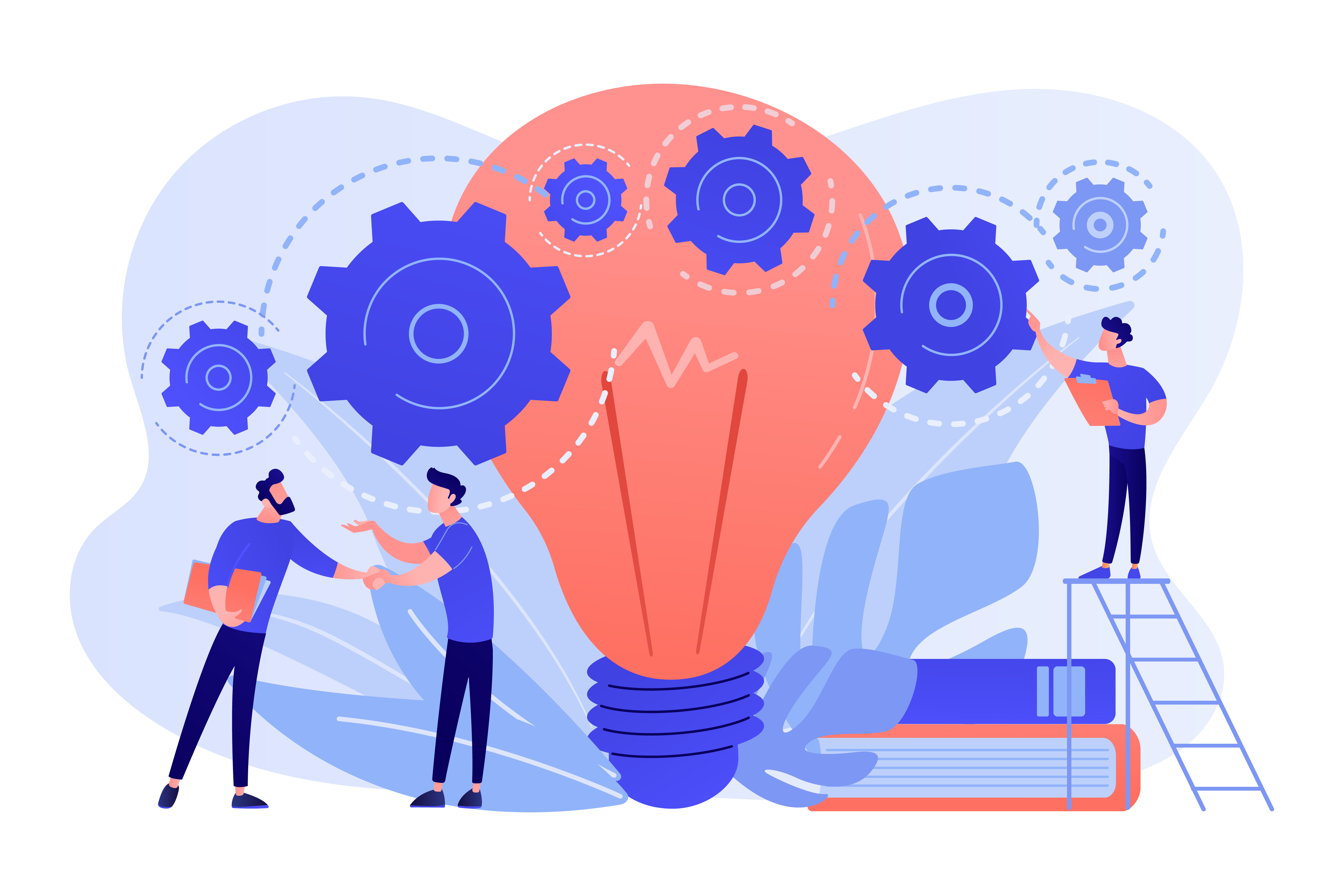
Despite having strong stored procedure functionality, Oracle and PostgreSQL differ greatly in syntax, error management, and debugging techniques. Heres a quick recap:
- Syntax: Oracle explicitly defines
IN OUT
,OUT
modes; PostgreSQL only usesIN
andOUT
. - Exception Handling: Oracle uses
SQLERRM
, while PostgreSQL relies onRAISE EXCEPTION
. - Debugging: Oracle has more integrated tools like
DBMS_OUTPUT
, whereas PostgreSQL depends onRAISE NOTICE
and logging.
By understanding these differences and using effective debugging techniques, you can become a more productive developer when working with Oracle or PostgreSQL stored procedures.
For deploying and managing databases efficiently, check out Nife.io, a cutting-edge platform that simplifies database deployment and scaling.
learn more about Database deployment Guide.