A Comprehensive Guide to Converting JSON to Structs in Go
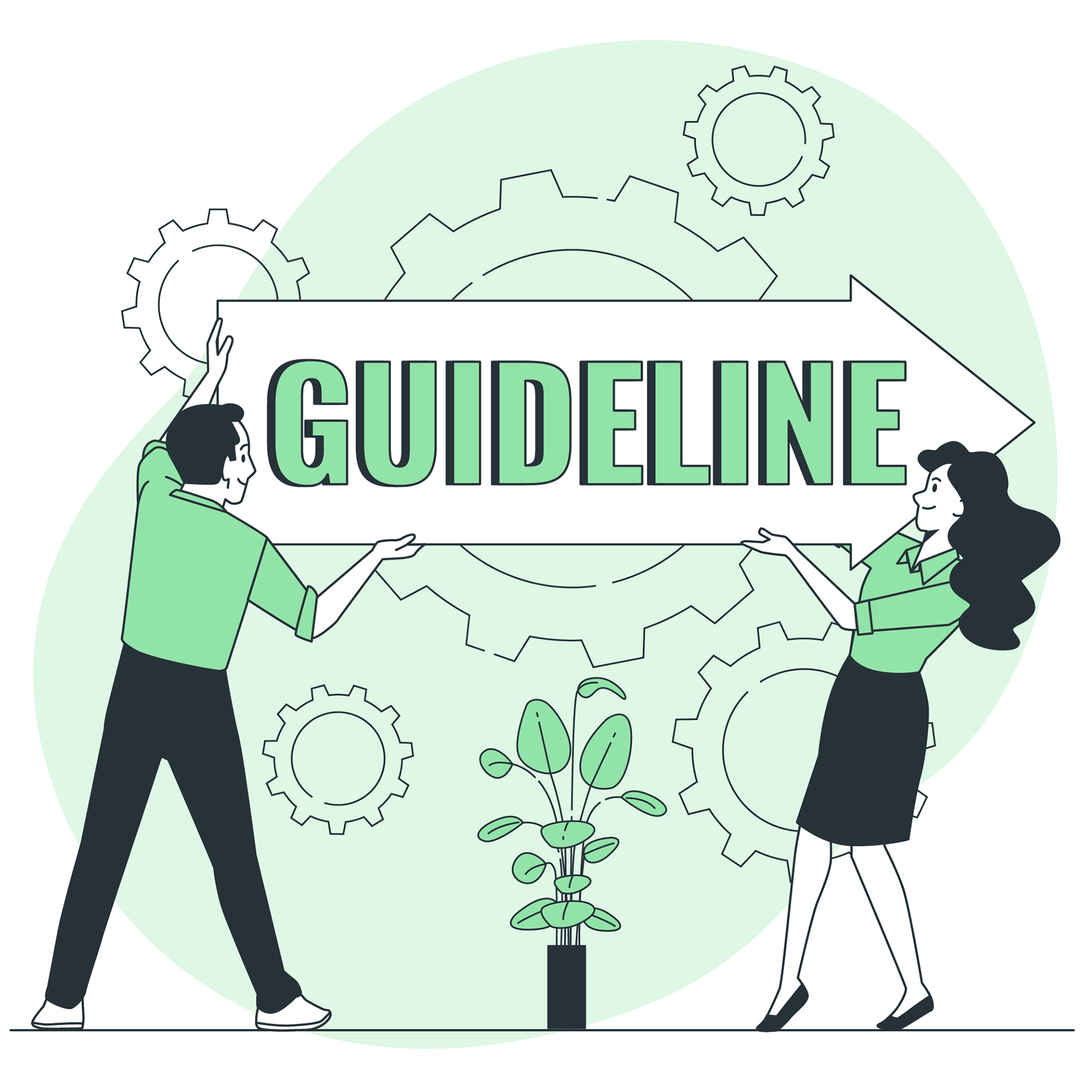
One of the most frequent jobs when working with Go (Golang) and JSON data is turning raw JSON data into a Go struct. Because structs offer a type-safe method of processing your JSON data, this procedure makes working with structured data in your Go applications simple.
We'll go over how to convert JSON to Go structs step-by-step in this blog article, emphasizing recommended practices, typical pitfalls, and things to consider as you go.
#
Why Use Go to Convert JSON to Structures?A string or raw byte slice is often what you get when you read a JSON file or retrieve data from an API. However, handling raw JSON data can be difficult. In your application, you want to be able to quickly obtain values, verify types, and work with data.
Transforming JSON into a Go struct allows you to:
- Ensure type safety: Avoid errors like interpreting an integer as a string because each field in the struct has a defined type.
- Simplify data access: Instead of constantly parsing JSON by hand, you can access values directly through struct fields.
- Improve error management: Go's type system can identify problems early in the compilation process rather than at runtime.
Let's start the process now!
#
Detailed Instructions for Converting JSON to Structure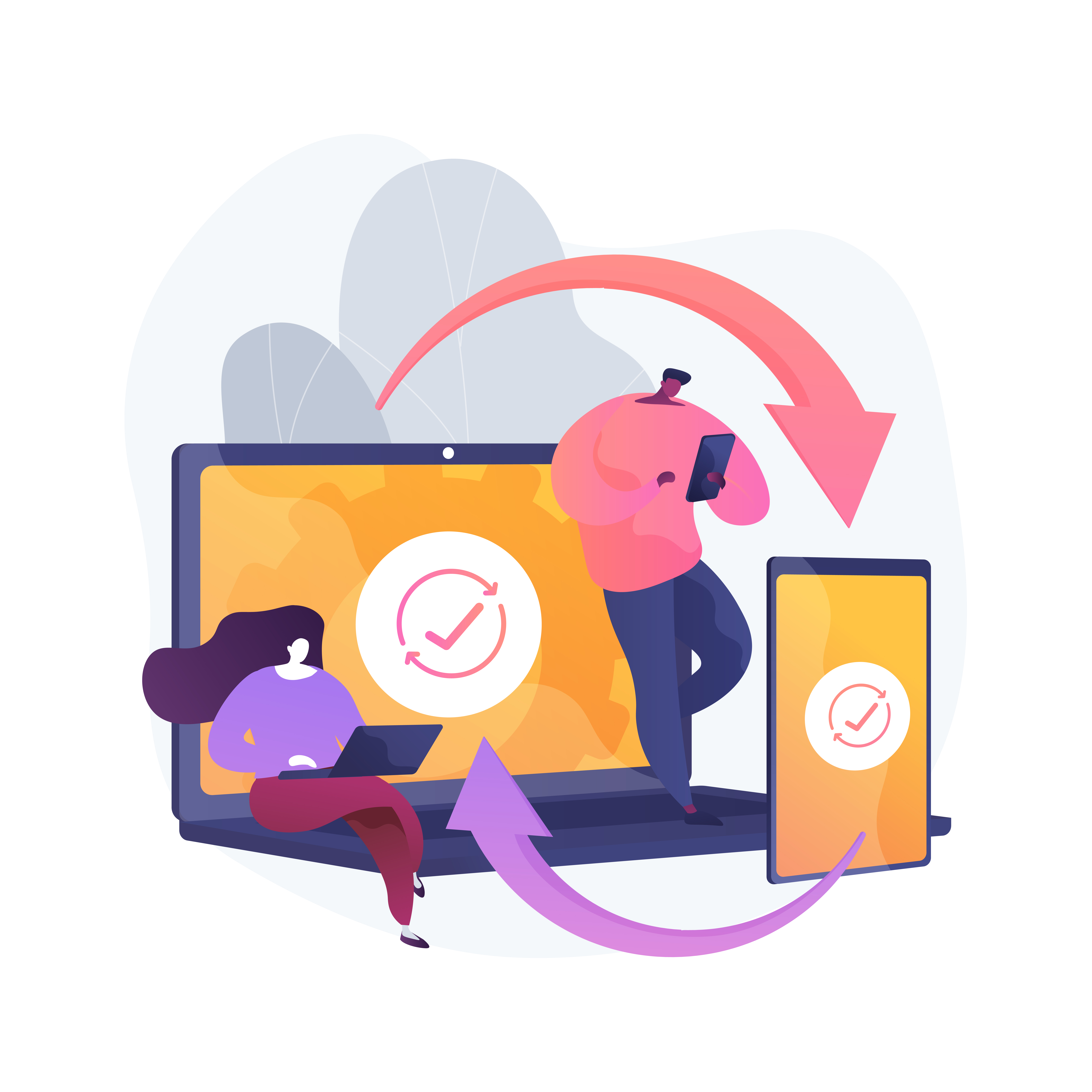
#
1. Establish Your StructureCreating a Go struct that corresponds to the JSON data's structure is the first step. The Go struct fields will be mapped to the appropriate JSON keys using struct tags, and each field in the struct should match a key in the JSON.
Here's a simple example. Suppose you have the following JSON:
The Go struct might look like this:
#
2. Unmarshal the JSON File into the Structure#
3. Managing JSON Objects That Are Nested#
4. Default Values and Optional Fields#
5. Managing Arrays#
6. Handling Unidentified Fields#
Best Practices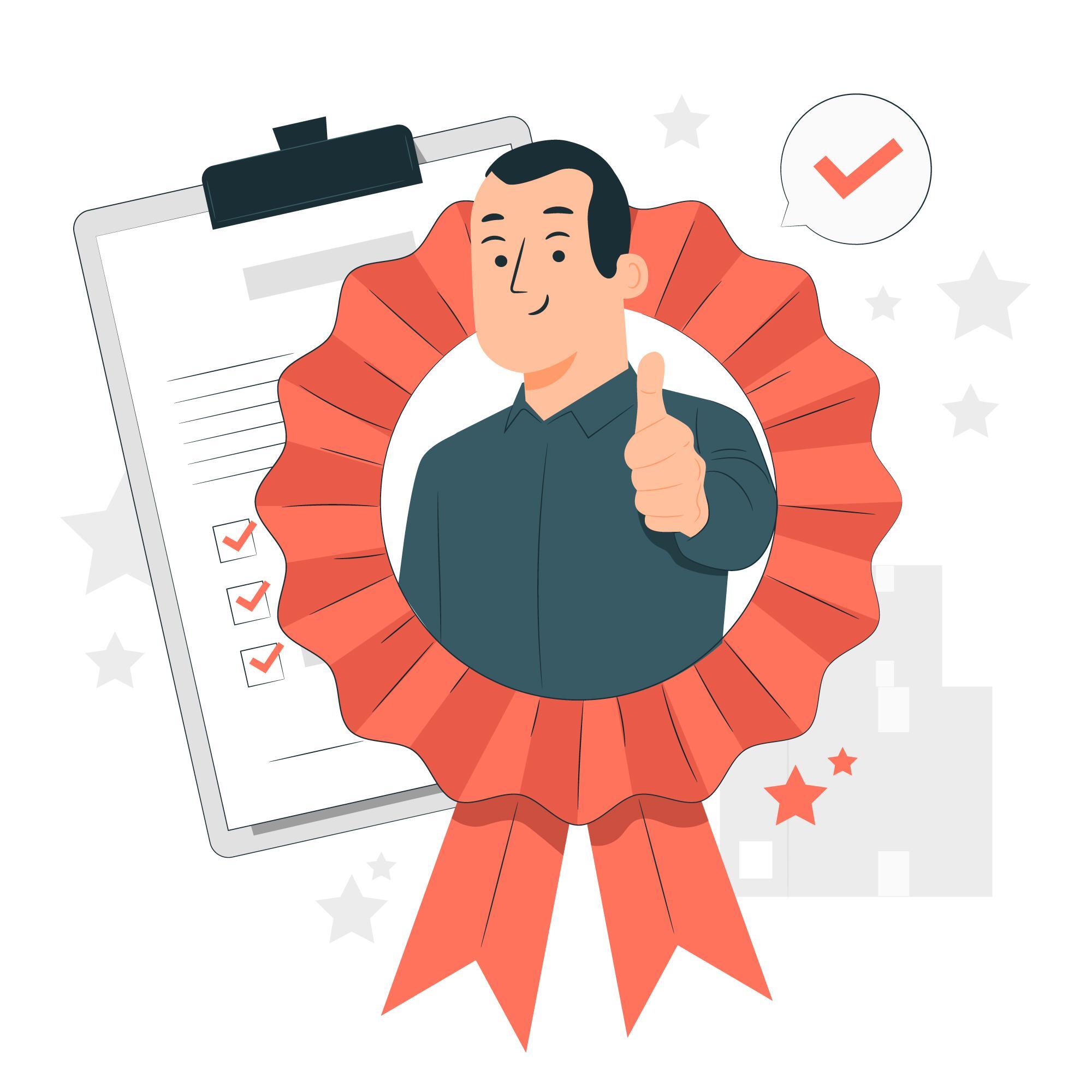
Align JSON keys with struct tags
- Match JSON keys correctly, e.g.,
json:"userName"
.
- Match JSON keys correctly, e.g.,
Avoid using
interface{}
unnecessarily- Prefer defined structs for type safety.
Use pointers for optional fields
- Helps differentiate between missing and empty fields.
Validate your JSON
- Ensure required fields and expected data types are present before unmarshalling.
Handle errors properly
- Always check and handle errors from
json.Unmarshal
.
- Always check and handle errors from
#
ConclusionConverting JSON to a Go struct is an essential skill for Go developers. It enhances type safety, simplifies data handling, and prevents errors. By following the steps and best practices outlined in this guide, you can efficiently process JSON data in your Go applications. Start transforming your JSON into structs today for a more structured, type-safe approach to data processing!
Deploy your Go application effortlessly at nife.io.
GitHub deployment, check out our documentation.