In the dynamic world of startups, effective release management plays a pivotal role in orchestrating the seamless delivery of product updates and enhancements, ensuring that innovation meets customer expectations while maintaining the agility essential for growth.
DevOps, a combination of "development" and "operations" in one word, is about everyone working together when a company works on app development and runs its computer systems. In simple terms, DevOps is a philosophy fostering enhanced communication and collaboration among various teams within an organization.
DevOps involves Using a step-by-step approach to develop software, Automate tasks and set up flexible infrastructure for deployment and upkeep.
It also fosters teamwork and trust between developers and system administrators and ensures that technology projects match business needs. DevOps can change how software is delivered, the services offered, job roles, IT tools, and established best practices.
But when you try to do all these things, you can avoid problems.
In this article, we'll talk about these problems and how to avoid them when running a startup and trying to be creative and innovative in software development. Along with that, we will discuss many other topics.
Role of DevOps in Enabling Efficient Release Management for Startups#
The Startup Challenge#
Developing software is a complex process requiring careful planning, expertise, and attention to detail. Starting a new company or developing a product from scratch is complex and lengthy. Here are some challenges that startups often face during their initial period:
Not Validating the Product#
It is important to give sufficient time to market research and customer development and develop a product that needs more demand to save time and resources.
Lack of a Clear Plan#
According to research, many startups need more time and financial resources to complete their projects earlier. A well-defined roadmap at the beginning of the product development process may allow progress and hinder the project's overall success.
Ignoring the UI/UX Design#
Many startups prioritize developing a technical solution without allocating sufficient resources or planning for their product design. Product design is creating a detailed plan or blueprint outlining a product's appearance and function before its development.
Marketing Strategy Not On Point#
Marketing activities and outcomes should be more noticed and prioritized than other business functions. This can be attributed to various reasons, such as a need to understand the value and impact of marketing, limited resources allocated to marketing efforts, or focusing on short-term results rather than long-term brand building.
Release Management for Startups#
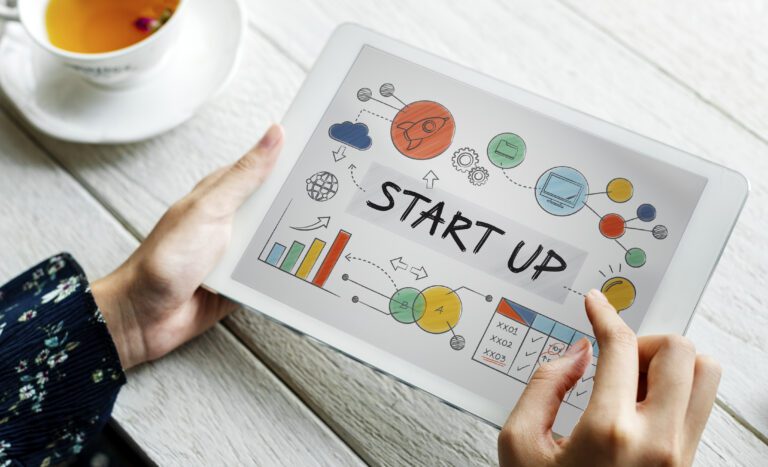
Can release management for startups make it easier? With proper management and collaboration with a team of experienced professionals, it is possible to achieve the desired outcome.
Effective release management is crucial for efficient work planning and achieving desired outcomes. By carefully managing the release management tools in DevOps, organizations can adequately prepare for anticipated results. For product consumers, quality support serves as a form of trust and a guarantee of receiving future enhancements and updates.
In addition, startups must invest in release management tools in DevOps to avoid expensive delays, unexpected bugs, or errors and ensure their organizational processes' smooth operation.
Understanding DevOps#
DevOps is like a teamwork approach to making software. It brings together the folks who create the software (dev) and those who manage it (ops). The goal is to make the whole process smoother and faster by eliminating obstacles and creating a team culture to improve things. In DevOps, we constantly put together new pieces of software, deliver them to users, and find ways to improve them. DevOps practices involve:
- Automating processes.
- Implementing infrastructure as code.
- Utilizing tools that enable efficient collaboration and communication between developers and operations personnel.
Consider the extended DevOps platform as a toolbox for making software. It's a collection of tools and technology that help people work together better when building software. These tools make it easier to collaborate and automate tasks from the beginning to the end of making software.
Using DevOps as a service can make things go faster and smoother when making software because it helps people work together better, simplifies how things get done, and makes it easier for everyone to talk to each other. Many teams start using DevOps using different tools, but these tools must be taken care of over time.
CI/CD#
Continuous Integration (CI) and Continuous Delivery (CD) are important tools in modern software development.
CI is about regularly compiling all the code changes from different developers in one place. This helps find and fix problems early.
The CD is about making it easy to release the software quickly and reliably. It automates the process of getting the software out to users.
Together, CI/CD forms an integral part of modern software development practices, enabling teams to deliver high-quality software faster. A purpose-built Continuous Integration/Continuous Deployment (CI/CD) platform is designed to optimize development time by enhancing an organization's productivity, improving efficiency, and streamlining workflows.
Key Benefits of DevOps for Startups#
Several strategies can be implemented for a business organization to gain a competitive edge and enhance efficiency in delivering optimal features to end-users within specified timelines.
1. Ensure faster deployment#
Many Saas release management companies aim at delivering updates and new features quickly to make customers happy. It also makes your company stronger in a tough market.
2. Stabilize the work environment#
Introducing new features or updates can sometimes cause tension and disrupt work. Use proven and extended DevOps platforms known for their effectiveness to create a more balanced and stable workspace.
3. Significant improvement in product quality#
When developers and operations teams work together, a product can be better. By collaborating and sharing ideas, they can make their work smoother and more efficient.
4. Automation in repetitive tasks leaves more room for innovation#
DevOps makes solving problems easier and helps teams work better together. It also makes fixing issues faster and smarter. Using automation to check for problems repeatedly gives the team more time to come up with new and better ideas.
5. Promotes agility in your business#
It is widely acknowledged that implementing agile practices in your business can provide a competitive advantage in the market. Adopting DevOps as a service allows businesses to achieve the scalability needed to drive transformation and growth.
6. Continuous delivery of software#
In DevOps, all departments share the responsibility for stability and new features. This speeds up software delivery compared to traditional methods.
7. Fast and reliable problem-solving techniques#
One of the primary benefits of DevOps is the ability to provide efficient and reliable solutions to technical errors in software management.
8. Transparency leads to high productivity#
Breaking down barriers and encouraging teamwork helps team members communicate easily and focus on their expertise. This has boosted productivity and efficiency in companies that use DevOps practices.
9. Minimal cost of production#
DevOps reduces departmental management and production expenses through effective collaboration by consolidating maintenance and updates under a unified umbrella.
Implementing DevOps in Startups#
How do you implement solutions for a startup with careful planning and execution? Here are some well-researched steps to consider:
Examining the current situation of the project.#
The first step is determining if we need to use the method. We also need to ensure our tech goals match a shared idea and that it helps our business. The aim is to find problems, set targets, explain DevOps jobs, and ensure everyone understands how things work in the project.
DevOps strategy formulation.#
After checking the current trend and what we want with DevOps as a service, we need a plan for using it in the project. This plan should make things work together, being faster, growing, staying safe, and using the best methods worldwide.
When planning a strategy, it's important to keep an eye on things, ensuring everyone knows what they're supposed to do in the DevOps team and their jobs. At this stage, organizations usually use Infrastructure as Code (IaC) to manage their work, make different tasks automatic, and get the right tools to do the job.
Utilization of containerization.#
This is a critical step in DevOps. It's about making different parts of the software work independently, not relying on the whole system. This helps you make changes fast, be more flexible, and make the software more dependable.
Implementation of CI/CD in the infrastructure.#
Setting up continuous integration and continuous delivery (CI/CD) pipelines is crucial in modern software development practices. These steps typically include software compilation, automated testing, production deployment, and efficiency tracking.
Test automation and QA-Dev alignment.#
Automated testing speeds up the delivery process, but not all tests must be done by machines. Some tests, like checking if the software works correctly, can still be done manually.
The Quality Assurance (QA) and Development (Devs) teams should work together to improve the quality of the product. This way, they can find and fix errors before launching the product.
Performance tracking and troubleshooting.#
Efficiency monitoring is a crucial aspect of the DevOps methodology, as it helps ensure transparency and accountability in the software development and operations process. Key Performance Indicators (KPIs) to measure and understand how we're doing. These KPIs serve as measurable metrics that provide insights into various aspects of the DevOps workflow, such as deployment frequency, lead time, change failure rate, and mean time to recover.
DevOps Tools#
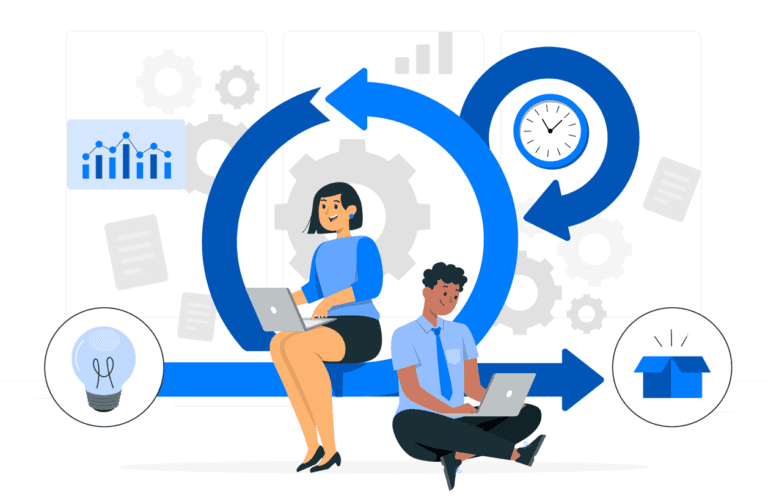
Choosing the right tools and extended DevOps platform is crucial for a successful DevOps strategy, and many popular and effective options are available. Let's look at the main tools.
- Configuration management: Puppet, Ansible, Salt, Chef.
- Virtual infrastructure: Amazon Web Services, VMware vCloud, Microsoft Azure.
- Continuous integration: Jenkins, GitLab, Bamboo, TeamCity, CircleCI.
- Continuous delivery: Docker, Maven.
- Continuous deployment: AWS CodeDeploy, Octopus Deploy, DeployBot, GitLab.
- Continuous testing: Selenium, Appium, Eggplant, Testsigma.
- Container management: Cloud Foundry, Red Hat OpenShift.
- Container orchestration: Kubernetes, Apache Mesos, OpenShift, Docker Swarm, Rancher.
Overcoming Challenges#
There are various challenges that startups may face while implementing DevOps. These include:
Environment Provisioning:#
It involves creating and managing the software's development, testing, and use. These environments are crucial for making software.
Solution:#
IaC allows developers to create and manage infrastructure resources, such as virtual machines, networks, and storage, using code-based configuration files.
Manual Testing:#
Manual testing, which most testing procedures rely on, takes time and may result in mistakes.
Solution:#
Implementing test automation, which uses technologies to automate the testing process, may help with this problem.
Lack of DevOps Center of Excellence:
With a DevOps center of excellence, your team might be able to implement DevOps efficiently, potentially causing issues like non-standardized processes, inconsistency, communication breakdowns, project delays, and higher expenses.
Solution:
To address this, consider creating a dedicated DevOps team as your center of excellence. An alternative is fostering a company-wide DevOps culture.
Test Data Management:
Managing test data is a significant challenge encountered during the implementation of DevOps. Effective test data management is crucial for ensuring accurate and efficient testing processes, as it helps mitigate potential issues and errors in software.
Solutions:
One effective approach to consider is using synthetic test data generation techniques. Another way is employing data masking, which obscures sensitive data during testing to safeguard it from exposure.
Security and Compliance:
Integrating security and compliance into every software delivery stage is crucial in DevOps. Neglecting this can lead to security breaches, regulatory breaches, and harm to your reputation.
Solution:
To tackle these issues, you can take a few steps. First, ensure that security and following the rules are a big part of how your DevOps team works together. Use automatic security tests to check for problems regularly, and also have regular checks to make sure you're following the rules. Additionally, you can use tools and methods like "security as code" and "infrastructure as code" to ensure everything is secure and follows the rules.
Case Studies#
Netflix, Amazon, and Etsy are good examples of how DevOps works well. Netflix made software delivery faster by always updating it, Amazon made its computer systems work well even when lots of people use them, and Etsy got things done quickly and helped customers more, making their businesses better.
Amazon#
Amazon, an online shopping company, faced big problems trying to guess how many people would visit their website. They had many servers, but about 40 percent of them still needed to be used, costing them money. This was especially troublesome during busy times like Christmas when many people shopped online and needed more servers.
Amazon made a smart move by using Amazon Web Services (AWS) to deal with these issues. They also started using DevOps methods, completely changing how they developed things. In just a year, they went from taking a long time to deploy stuff to being super fast, with an average deployment time of 11.7 seconds. This shows how quick and agile they became.
Netflix#
It was a big leap into the unknown when Netflix switched from sending DVDs by mail to streaming movies online. They had a massive computer system in the cloud, but few tools were available to manage it. So, Netflix decided to use free software made by lots of volunteers.
They created a bunch of automated tools called the Simian Army. These tools helped them constantly test their system, ensuring it worked well. By finding and fixing problems before they could affect viewers, Netflix ensured that watching movies online was smooth and trouble-free.
Netflix also made a big deal out of using automation and free software.
Conclusion#
Adopting DevOps may seem challenging, but you can make the most of it by recognizing and tackling the obstacles. From setting up environments to dealing with training gaps, you can overcome these issues using techniques like fostering the right culture, improving communication, automating tasks, and working together as a team.
Focusing on frequent iterative releases, many release management tools in DevOps help software projects go through their defined lifecycle phases.
Also, adopting SaaS release management techniques helps companies improve their standing in the market.
We have seen many companies like Amazon, Netflix, etc., improve their system after the implementation of DevOps.