Migrating from Create React App (CRA) to Next.js: A Step-by-Step Guide
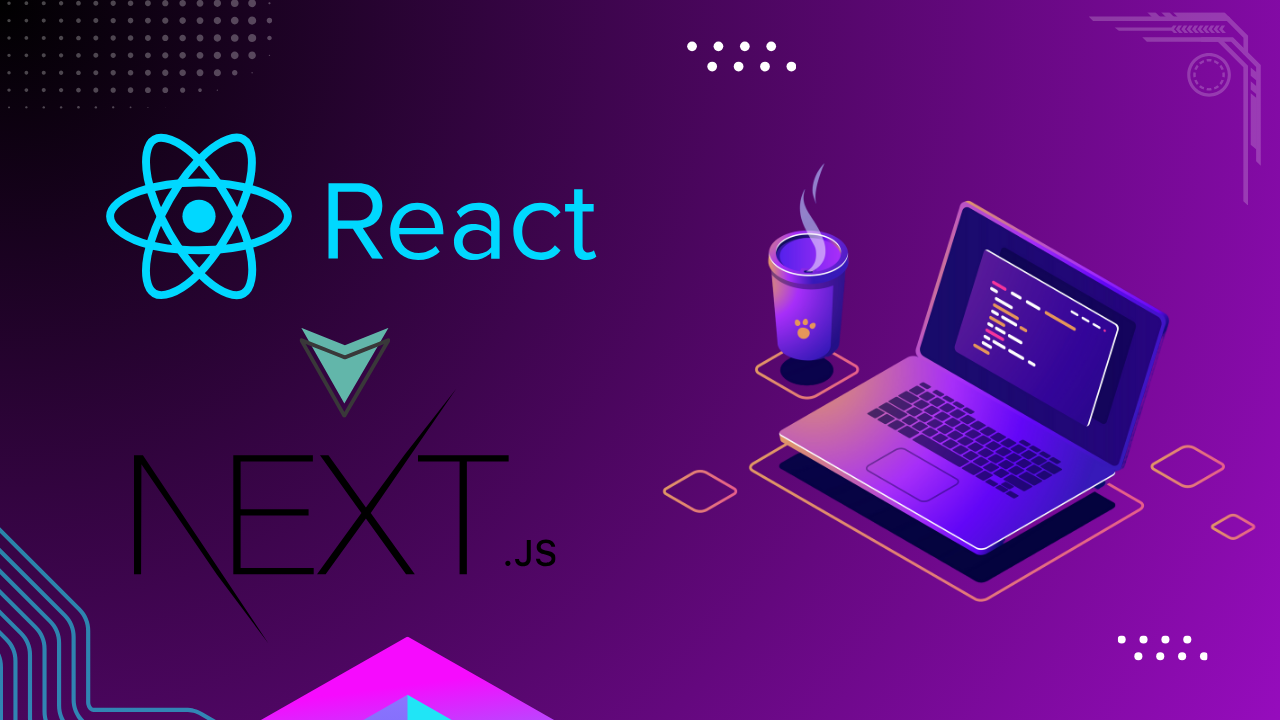
Next.js has been a popular choice among React developers because to its built-in features like as server-side rendering (SSR), static site generation (SSG), and a strong emphasis on performance and scalability. If you already have a project developed with Create React App (CRA) and want to migrate to Next.js, this guide will bring you through the process step by step.
#
Why Migrate from CRA to Next.js?Before diving into the migration process, let's explore the benefits of Next.js over CRA:
- Improved Performance:SSR and SSG increase page load time and SEO.
- Built-in Routing: Next.js provides file-based routing, which eliminates the requirement for libraries such as React Router.
- API Routes: Create serverless functions from within your app.
- Optimized Bundling: Next.js offers improved tree-shaking and code splitting.
Learn more about Next.js features.
#
Step 1: Set Up the Next.js ProjectStart by creating a new Next.js project:
If you use TypeScript in your CRA project, you can enable it in Next.js by renaming files to '.tsx' and installing the required dependencies:
#
Step 2: Move CRA Files to Next.jssrc
Files#
1. Copy Copy all files from the src
folder in your CRA project to the pages
or components
folder in your Next.js project. Organize them logically:
- Place React components in a
components
folder. - Place page-level components in the
pages
folder.
#
2. Transfer Static FilesMove files from the public
directory of CRA to the public
directory in Next.js.
index.js
#
3. Remove Next.js uses pages/index.js
as the default entry point. Rename or move your App.js
content to pages/index.js
.
#
Step 3: Update RoutingNext.js employs file-based routing, so you don't require a routing package like React Router. Replace React Router routes with this file structure:
#
1. Update Route LogicIn CRA:
In Next.js, create corresponding files:
#
2. Update NavigationReplace <Link>
from React Router with Next.js's <Link>
:
Read more about Next.js routing.
#
Step 4: Update StylesIf you're using CSS or Sass, ensure styles are compatible with Next.js:
#
1. Global StylesMove CRA's index.css
to styles/globals.css
in Next.js.
Import it in pages/_app.js
:
#
2. CSS ModulesNext.js supports CSS Modules out of the box. Rename CSS files to [ComponentName].module.css
and import them directly into the component.
#
Step 5: Update API CallsNext.js supports server-side logic via API routes. If your CRA app relies on a separate backend or makes API calls, you can:
#
1. Migrate API CallsMove server-side logic to pages/api
. For example:
#
2. Update Client-Side FetchesUpdate fetch URLs to point to the new API routes or external APIs.
#
Step 6: Optimize for SSR and SSGNext.js provides several data-fetching methods. Replace CRA's useEffect
with appropriate Next.js methods:
#
1. Static Site Generation (SSG)#
2. Server-Side Rendering (SSR)#
Step 7: Install Required DependenciesNext.js requires some specific dependencies that may differ from CRA:
- Install any missing packages:
- Install additional packages if you used specific libraries in CRA (e.g., Axios, Redux, Tailwind CSS).
#
Step 8: Test the Application- Run the development server:
- Check the console and fix any errors or warnings.
- Test all pages and routes to ensure the migration was successful.
#
Step 9: Deploy the Next.js AppNext.js simplifies deployment with platforms like Oikos by Nife:
- Push your project to a Git repository (e.g., GitHub).
- Build your Next.js app locally.
- Upload your build app from the Oikos dashboard and deploy it.
Learn more about Site Deployment.
#
ConclusionMigrating from CRA to Next.js may appear difficult, but by following these steps, you may fully benefit from Next.js' advanced capabilities and performance optimizations. Your migration will go smoothly and successfully if you plan ahead of time and test thoroughly.